Glyphs.app Python Scripting API Documentation¶
This is the documentation for the Python Scripting API for Glyphs.app (glyphsapp.com)
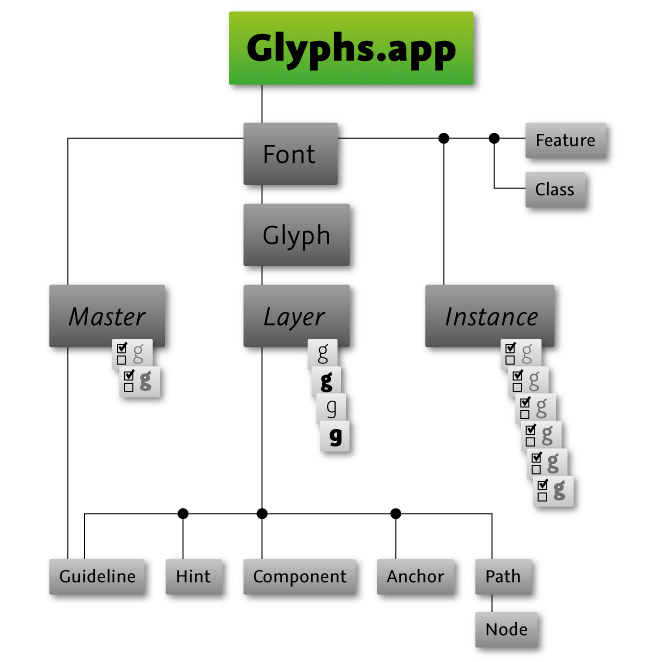
About this Document¶
This document covers all methods that are implemented in the Python wrapper. There are many more functions and objects available via the PyObjC bridge. For more details, please have a look at the Core part of the documentation.
Changes in the API¶
These changes could possibly break your code, so you need to keep track of them. Please see GSApplication.versionNumber
for how to check for the app version in your code. Really, read it. There’s a catch.
Major Changes in 2.5¶
- Add pointPen capabilities to GSPathPen
- Add setter for
GSFont.selection()
- Add
GSFont.axes
,GSFontMaster.axes
andGSInstance.axes
- Add
GSLayer.componentLayer
- The
GSLayer
the component is pointing to. This is read-only. In order to change the referenced base glyph, setGSComponent.componentName
to the new glyph name. For Smart Components, the componentLayer contains the interpolated result.- Add
GSInstance.generate(UFO)()
- Add
GSFont.export()
- This allows to export Variable fonts
- Add
GSGlyph.unicodes
Major Changes in 2.3¶
-
*.bezierPath
We’ve created a distinct .bezierPath
attribute for various objects (paths, components, etc.) to use to draw in plug-ins, over-writing the previous (and never documented) .bezierPath() method (from the Python-ObjC-bridge) by the same name that handed down an NSBezierPath object.
Old: .bezierPath()
New: .bezierPath
GSApplication
¶
The mothership. Everything starts here.
print(Glyphs)
<Glyphs.app>
-
class
GSApplication
¶ Properties
Functions
Properties
-
font
¶
Returns: The active GSFont
object or None.Return type: GSFont
# topmost open font font = Glyphs.font
-
fonts
¶
Returns: All open fonts
.# access all open fonts for font in Glyphs.fonts: print(font.familyName) # add a font font = GSFont() font.familyName = "My New Fonts" Glyphs.fonts.append(font)
-
reporters
¶
List of available reporter plug-ins (same as bottom section in the ‘View’ menu). These are the actual objects. You can get hold of their names using object.__class__.__name__.
Also see
GSApplication.activateReporter()
andGSApplication.deactivateReporter()
methods below to activate/deactivate them.# List of all reporter plug-ins print(Glyphs.reporters) # Individual plug-in class names for reporter in Glyphs.reporters: print(reporter.__class__.__name__) # Activate a plugin Glyphs.activateReporter(Glyphs.reporters[0]) # by object Glyphs.activateReporter('GlyphsMasterCompatibility') # by class name
New in version 2.3.
-
activeReporters
¶
List of activated reporter plug-ins.
New in version 2.3.
-
filters
¶
List of available filters (same as ‘Filter’ menu). These are the actual objects.
Below sample code shows how to get hold of a particular filter and use it. You invoke it using the processFont_withArguments_() function for old plugins, or the filter() function for newer plugins. As arguments you use the list obtained by clicking on ‘Copy Custom Parameter’ button in the filter’s dialog (gear icon) and convert it to a list. In the include option you can supply a comma-separated list of glyph names. Here’s a catch: old plugins will only run on the first layer of a glyph, because the function processFont_withArguments_() was designed to run on instances upon export that have already been reduced to one layer. You can work around that by changing the order of the layers, then changing them back (not shown in the sample code).
# Helper function to get filter by its class name def filter(name): for filter in Glyphs.filters: if filter.__class__.__name__ == name: return filter # Get the filter offsetCurveFilter = filter('GlyphsFilterOffsetCurve') # Run the filter (old plugins) # The arguments came from the 'Copy Custom Parameter' as: # Filter = "GlyphsFilterOffsetCurve;10;10;1;0.5;" offsetCurveFilter.processFont_withArguments_(font, ['GlyphsFilterOffsetCurve', '10', '10', '1', '0.5', 'include:%s' % glyph.name]) # If the plugin were a new filter, the same call would look like this: # (run on a specific layer, not the first layer glyphs in the include-list) # The arguments list is a dictionary with either incrementing integers as keys or names (as per 'Copy Custom Parameter' list) offsetCurveFilter.filter(layer, False, {0: 10, 1: 10, 2: 1, 3: 0.5})
New in version After: 2.4.2
-
defaults
¶
A dict like object for storing preferences. You can get and set key-value pairs.
Please be careful with your keys. Use a prefix that uses the reverse domain name. e.g. “com.MyName.foo.bar”.
# Check for whether or not a preference exists, because has_key() doesn't work in this PyObjC-brigde if Glyphs.defaults["com.MyName.foo.bar"] is None: # do stuff # Get and set values value = Glyphs.defaults["com.MyName.foo.bar"] Glyphs.defaults["com.MyName.foo.bar"] = newValue # Remove value # This will restore the default value del(Glyphs.defaults["com.MyName.foo.bar"])
-
scriptAbbreviations
¶
A dictionary with script name to abbreviation mapping, e.g., ‘arabic’: ‘arab’
Return type: dict` -
scriptSuffixes
¶
A dictionary with glyphs name suffixes for scripts and their respective script names, e.g., ‘cy’: ‘cyrillic’
Return type: dict` -
languageScripts
¶
A dictionary with language tag to script tag mapping, e.g., ‘ENG’: ‘latn’
Return type: dict` -
languageData
¶
A list of dictionaries with more detailed language informations.
Return type: list` -
unicodeRanges
¶
Names of unicode ranges.
Return type: list` -
editViewWidth
¶
New in version 2.3.
Width of glyph Edit view. Corresponds to the “Width of editor” setting from the Preferences.
Type: int -
handleSize
¶
New in version 2.3.
Size of Bezier handles in Glyph Edit view. Possible value are 0–2. Corresponds to the “Handle size” setting from the Preferences.
To use the handle size for drawing in reporter plugins, you need to convert the handle size to a point size, and divide by the view’s scale factor. See example below.
# Calculate handle size handSizeInPoints = 5 + Glyphs.handleSize * 2.5 # (= 5.0 or 7.5 or 10.0) scaleCorrectedHandleSize = handSizeInPoints / Glyphs.font.currentTab.scale # Draw point in size of handles point = NSPoint(100, 100) NSColor.redColor.set() rect = NSRect((point.x - scaleCorrectedHandleSize * 0.5, point.y - scaleCorrectedHandleSize * 0.5), (scaleCorrectedHandleSize, scaleCorrectedHandleSize)) bezierPath = NSBezierPath.bezierPathWithOvalInRect_(rect) bezierPath.fill()
Type: int -
versionString
¶
New in version 2.3.
String containing Glyph.app’s version number. May contain letters also, like ‘2.3b’. To check for a specific version, use .versionNumber below.
Type: string -
versionNumber
¶
New in version 2.3.
Glyph.app’s version number. Use this to check for version in your code.
Here’s the catch: Since we only added this versionNumber attribute in Glyphs v2.3, it is not possible to use this attribute to check for versions of Glyphs older than 2.3. We’re deeply sorry for this inconvenience. Development is a slow and painful process.
So you must first check for the existence of the versionNumber attribute like so:
# Code valid for Glyphs.app v2.3 and above: if hasattr(Glyphs, 'versionNumber') and Glyphs.versionNumber >= 2.3: # do stuff # Code for older versions else: # do other stuff
Type: float -
buildNumber
¶
New in version 2.3.
Glyph.app’s build number.
Especially if you’re using preview builds, this number may be more important to you than the version number. The build number increases with every released build and is the most significant evidence of new Glyphs versions, while the version number is set arbitrarily and stays the same until the next stable release.
Type: int New in version 2.3.1-910.
Add menu items to Glyphs’ main menus.
Following constants for accessing the menus are defined:
APP_MENU
,FILE_MENU
,EDIT_MENU
,GLYPH_MENU
,PATH_MENU
,FILTER_MENU
,VIEW_MENU
,SCRIPT_MENU
,WINDOW_MENU
,HELP_MENU
def doStuff(sender): # do stuff newMenuItem = NSMenuItem('My menu title', doStuff) Glyphs.menu[EDIT_MENU].append(newMenuItem)
Functions
-
open
(Path[, showInterface=True])¶
Opens a document
Parameters: - Path (str) – The path where the document is located.
- showInterface (bool) – If a document window should be opened. Default: True
Returns: The opened document object or None.
Return type: -
showMacroWindow
()¶
Opens the macro window
-
clearLog
()¶
Deletes the content of the console in the macro window
-
showGlyphInfoPanelWithSearchString
(String)¶
Shows the Glyph Info window with a preset search string
Parameters: String – The search term -
glyphInfoForName
(String)¶
Generates
GSGlyphInfo
object for a given glyph name.Parameters: - String – Glyph name
- font – if you add a font, and the font has a local glyph info, it will be used instead of the global info data.
Returns: -
glyphInfoForUnicode
(Unicode)¶
Generates
GSGlyphInfo
object for a given hex unicode.Parameters: - String – Hex unicode
- font – if you add a font, and the font has a local glyph info, it will be used instead of the global info data.
Returns: -
niceGlyphName
(Name)¶
Converts glyph name to nice, human-readable glyph name (e.g. afii10017 or uni0410 to A-cy)
Parameters: - string – glyph name
- font – if you add a font, and the font has a local glyph info, it will be used instead of the global info data.
Returns: string
-
productionGlyphName
(Name)¶
Converts glyph name to production glyph name (e.g. afii10017 or A-cy to uni0410)
Parameters: - string – glyph name
- font – if you add a font, and the font has a local glyph info, it will be used instead of the global info data.
Returns: string
-
ligatureComponents
(String)¶
If defined as a ligature in the glyph database, this function returns a list of glyph names that this ligature could be composed of.
Parameters: - string – glyph name
- font – if you add a font, and the font has a local glyph info, it will be used instead of the global info data.
Returns: list
print(Glyphs.ligatureComponents('allah-ar')) ( "alef-ar", "lam-ar.init", "lam-ar.medi", "heh-ar.fina" )
-
addCallback
(function, hook)¶
New in version 2.3.
Add a user-defined function to the glyph window’s drawing operations, in the foreground and background for the active glyph as well as in the inactive glyphs.
The function names are used to add/remove the functions to the hooks, so make sure to use unique function names.
Your function needs to accept two values: layer which will contain the respective
GSLayer
object of the layer we’re dealing with and info which is a dictionary and contains the value Scale (for the moment).For the hooks these constants are defined: DRAWFOREGROUND, DRAWBACKGROUND, DRAWINACTIVE, DOCUMENTWASSAVED, DOCUMENTOPENED, TABDIDOPEN, TABWILLCLOSE, UPDATEINTERFACE, MOUSEMOVED. For more information check the constants section.
def drawGlyphIntoBackground(layer, info): # Due to internal Glyphs.app structure, we need to catch and print exceptions # of these callback functions with try/except like so: try: # Your drawing code here NSColor.redColor().set() layer.bezierPath.fill() # Error. Print exception. except: import traceback print(traceback.format_exc()) # add your function to the hook Glyphs.addCallback(drawGlyphIntoBackground, DRAWBACKGROUND)
-
removeCallback
(function)¶
New in version 2.3.
Remove the function you’ve previously added.
# remove your function to the hook Glyphs.removeCallback(drawGlyphIntoBackground)
-
redraw
()¶
Redraws all Edit views and Preview views.
-
showNotification
(title, message)¶
Shows the user a notification in Mac’s Notification Center.
Glyphs.showNotification('Export fonts', 'The export of the fonts was successful.')
-
localize
(localization)¶
New in version 2.3.
Return a string in the language of Glyphs.app’s UI locale, which must be supplied as a dictionary using language codes as keys.
The argument is a dictionary in the languageCode: translatedString format.
You don’t need to supply strings in all languages that the Glyphs.app UI supports. A subset will do. Just make sure that you add at least an English string to default to next to all your other translated strings. Also don’t forget to mark strings as unicode strings (u’öäüß’) when they contain non-ASCII content for proper encoding, and add a # encoding: utf-8 to the top of all your .py files.
Tip: You can find Glyphs’ localized languages here Glyphs.defaults[“AppleLanguages”].
# encoding: utf-8 print(Glyphs.localize({ 'en': 'Hello World', 'de': u'Hallöle Welt', 'fr': 'Bonjour tout le monde', 'es': 'Hola Mundo', })) # Given that your Mac’s system language is set to German # and Glyphs.app UI is set to use localization (change in preferences), # it will print: > Hallöle Welt
-
activateReporter
(reporter)¶
New in version 2.3.
Activate a reporter plug-in by its object (see Glyphs.reporters) or class name.
Glyphs.activateReporter('GlyphsMasterCompatibility')
-
deactivateReporter
(reporter)¶
New in version 2.3.
Deactivate a reporter plug-in by its object (see Glyphs.reporters) or class name.
Glyphs.deactivateReporter('GlyphsMasterCompatibility')
-
font
The active
GSFont
Type: list -
GSFont
¶
Implementation of the font object. This object is host to the masters
used for interpolation. Even when no interpolation is involved, for the sake of object model consistency there will still be one master and one instance representing a single font.
Also, the glyphs
are attached to the Font object right here, not one level down to the masters. The different masters’ glyphs are available as layers
attached to the glyph objects which are attached here.
-
class
GSFont
¶ Properties
Functions
save()
close()
show()
disableUpdateInterface()
enableUpdateInterface()
kerningForPair()
setKerningForPair()
removeKerningForPair()
newTab()
updateFeatures()
compileFeatures()
Properties
-
parent
¶
Returns the internal NSDocument document. Read-only.
Type: NSDocument -
masters
¶
Collection of
GSFontMaster
objects.Type: list -
instances
¶
Collection of
GSInstance
objects.Type: list -
axes
¶
- a list of dicts:
- {“Name”:”Weight”, “Tag”:”wght”}
Type: list New in version 2.5.
-
glyphs
¶
Collection of
GSGlyph
objects. Returns a list, but you may also call glyphs using index or glyph name or character (as of v2.4) as key.# Access all glyphs for glyph in font.glyphs: print(glyph) <GSGlyph "A" with 4 layers> <GSGlyph "B" with 4 layers> <GSGlyph "C" with 4 layers> ... # Access one glyph print(font.glyphs['A']) <GSGlyph "A" with 4 layers> # Access a glyph by character (new in v2.4.1) print(font.glyphs[u'Ư']) <GSGlyph "Uhorn" with 4 layers> # Access a glyph by unicode (new in v2.4.1) print(font.glyphs['01AF']) <GSGlyph "Uhorn" with 4 layers> # Add a glyph font.glyphs.append(GSGlyph('adieresis')) # Duplicate a glyph under a different name newGlyph = font.glyphs['A'].copy() newGlyph.name = 'A.alt' font.glyphs.append(newGlyph) # Delete a glyph del(font.glyphs['A.alt'])
Type: list, dict -
classes
¶
Collection of
GSClass
objects, representing OpenType glyph classes.Type: list # add a class font.classes.append(GSClass('uppercaseLetters', 'A B C D E')) # access all classes for class in font.classes: print(class.name) # access one class print(font.classes['uppercaseLetters'].code) # delete a class del(font.classes['uppercaseLetters'])
-
features
¶
Collection of
GSFeature
objects, representing OpenType features.Type: list # add a feature font.features.append(GSFeature('liga', 'sub f i by fi;')) # access all features for feature in font.features: print(feature.code) # access one feature print(font.features['liga'].code) # delete a feature del(font.features['liga'])
-
featurePrefixes
¶
Collection of
GSFeaturePrefix
objects, containing stuff that needs to be outside of the OpenType features.Type: list # add a prefix font.featurePrefixes.append(GSFeaturePrefix('LanguageSystems', 'languagesystem DFLT dflt;')) # access all prefixes for prefix in font.featurePrefixes: print(prefix.code) # access one prefix print(font.featurePrefixes['LanguageSystems'].code) # delete del(font.featurePrefixes['LanguageSystems'])
-
copyright
¶
Type: unicode -
designer
¶
Type: unicode -
designerURL
¶
Type: unicode -
manufacturer
¶
Type: unicode -
manufacturerURL
¶
Type: unicode -
versionMajor
¶
Type: int -
versionMinor
¶
Type: int -
date
¶
Type: NSDate print(font.date) 2015-06-08 09:39:05 +0000 # set date to now font.date = NSDate.date()
-
familyName
¶
Family name of the typeface.
Type: unicode -
upm
¶
Units per Em
Type: int -
note
¶
Type: unicode -
kerning
¶
A multi-level dictionary. The first level’s key is the
GSFontMaster.id
(each master has its own kerning), the second level’s key is theGSGlyph.id
or class id (@MMK_L_XX) of the first glyph, the third level’s key is a glyph id or class id (@MMK_R_XX) for the second glyph. The values are the actual kerning values.To set a value, it is better to use the method
GSFont.setKerningForPair()
. This ensures a better data integrity (and is faster).Type: dict -
userData
¶
A dictionary to store user data. Use a unique key and only use objects that can be stored in a property list (string, list, dict, numbers, NSData) otherwise the data will not be recoverable from the saved file.
Type: dict # set value font.userData['rememberToMakeCoffee'] = True # delete value del font.userData['rememberToMakeCoffee']
-
disablesNiceNames
¶
Corresponds to the “Don’t use nice names” setting from the Font Info dialog.
Type: bool -
customParameters
¶
The custom parameters. List of
GSCustomParameter
objects. You can access them by name or by index.# access all parameters for parameter in font.customParameters: print(parameter) # set a parameter font.customParameters['trademark'] = 'ThisFont is a trademark by MyFoundry.com' # delete a parameter del(font.customParameters['trademark'])
Type: list, dict -
grid
¶
New in version 2.3.
Corresponds to the “Grid spacing” setting from the Info dialog.
Type: int -
gridSubDivisions
¶
New in version 2.3.
Corresponds to the “Grid sub divisions” setting from the Info dialog.
Type: int -
gridLength
¶
Ready calculated size of grid for rounding purposes. Result of division of grid with gridSubDivisions.
Type: float -
disablesAutomaticAlignment
¶
Type: bool -
keyboardIncrement
¶
distance of movement by arrow keys. Default:1
Type: float New in version 2.3.1.
-
selection
¶
New in version 2.3.
Returns a list of all selected glyphs in the Font View.
Type: list -
selectedLayers
¶
Returns a list of all selected layers in the active tab.
If a glyph is being edited, it will be the only glyph returned in this list. Otherwise the list will contain all glyphs selected with the Text tool.
Type: list -
selectedFontMaster
¶
Returns the active master (selected in the toolbar).
Type: GSFontMaster
-
masterIndex
¶
Returns the index of the active master (selected in the toolbar).
Type: int -
currentText
¶
The text of the current Edit view.
Unencoded and none ASCII glyphs will use a slash and the glyph name. (e.g: /a.sc). Setting unicode strings works.
Type: unicode -
tabs
¶
List of open Edit view tabs in UI, as list of
GSEditViewController
objects.# open new tab with text font.newTab('hello') # access all tabs for tab in font.tabs: print(tab) # close last tab font.tabs[-1].close()
Type: list -
fontView
¶
:type GSFontViewController
-
currentTab
¶
Active Edit view tab.
Type: GSEditViewController
-
filepath
¶
On-disk location of GSFont object.
Type: unicode -
tool
¶
Name of tool selected in toolbar.
For available names including third-party plug-ins that come in the form of selectable tools, see GSFont.tools below.
font.tool = 'SelectTool' # Built-in tool font.tool = 'GlyphsAppSpeedPunkTool' # Third party plug-in
Type: string New in version 2.3.
-
tools
¶
Prints a list of available tool names, including third-party plug-ins.
Type: list, string New in version 2.3.
-
appVersion
¶
returns the version that the file was last saved
New in version 2.5.
Functions
-
save
([filePath])¶
Saves the font. if no path is given, it saves to the existing location.
Parameters: filePath (str) – Optional file path -
close
([ignoreChanges = False])¶
Closes the font.
Parameters: ignoreChanges (bool) – Optional. Ignore changes to the font upon closing -
disableUpdateInterface
()¶
Disables interface updates and thus speeds up glyph processing. Call this before you do big changes to the font, or to its glyphs. Make sure that you call Font.enableUpdateInterface() when you are done.
-
enableUpdateInterface
()¶
This re-enables the interface update. Only makes sense to call if you have disabled it earlier.
-
show
()¶
Makes font visible in the application, either by bringing an already open font window to the front or by appending a formerly invisible font object (such as the result of a copy() operation) as a window to the application.
New in version 2.4.1.
-
kerningForPair
(fontMasterId, leftKey, rightKey[, direction = LTR])¶
This returns the kerning value for the two specified glyphs (leftKey or rightKey is the glyph name) or a kerning group key (@MMK_X_XX).
Parameters: - fontMasterId (str) – The id of the FontMaster
- leftKey (str) – either a glyph name or a class name
- rightKey (str) – either a glyph name or a class name
- direction (str) – optional writing direction (see Constants). Default is LTR.
Returns: The kerning value
Return type: float
# print(kerning between w and e for currently selected master) font.kerningForPair(font.selectedFontMaster.id, 'w', 'e') -15.0 # print(kerning between group T and group A for currently selected master) # ('L' = left side of the pair and 'R' = left side of the pair) font.kerningForPair(font.selectedFontMaster.id, '@MMK_L_T', '@MMK_R_A') -75.0 # in the same font, kerning between T and A would be zero, because they use group kerning instead. font.kerningForPair(font.selectedFontMaster.id, 'T', 'A') 9.22337203685e+18 # (this is the maximum number for 64 bit. It is used as an empty value)
-
setKerningForPair
(fontMasterId, leftKey, rightKey, value[, direction = LTR])¶
This sets the kerning for the two specified glyphs (leftKey or rightKey is the glyphname) or a kerning group key (@MMK_X_XX).
Parameters: - fontMasterId (str) – The id of the FontMaster
- leftKey (str) – either a glyph name or a class name
- rightKey (str) – either a glyph name or a class name
- value (float) – kerning value
- direction (str) – optional writing direction (see Constants). Default is LTR.
# set kerning for group T and group A for currently selected master # ('L' = left side of the pair and 'R' = left side of the pair) font.setKerningForPair(font.selectedFontMaster.id, '@MMK_L_T', '@MMK_R_A', -75)
-
removeKerningForPair
(FontMasterId, LeftKey, RightKey)¶
Removes the kerning for the two specified glyphs (LeftKey or RightKey is the glyphname) or a kerning group key (@MMK_X_XX).
Parameters: - FontMasterId (str) – The id of the FontMaster
- LeftKey (str) – either a glyph name or a class name
- RightKey (str) – either a glyph name or a class name
# remove kerning for group T and group A for all masters # ('L' = left side of the pair and 'R' = left side of the pair) for master in font.masters: font.removeKerningForPair(master.id, '@MMK_L_T', '@MMK_R_A')
-
newTab
([tabText])¶
Opens a new tab in the current document window, optionally with text, and return that tab object
Parameters: tabText – Text or glyph names escaped with ‘/’ # open new tab font.newTab('abcdef') # or tab = font.newTab('abcdef') print(tab)
-
updateFeatures
()¶
Updates all OpenType features and classes at once, including generating necessary new features and classes. Equivalent to the “Update” button in the features panel. This already includes the compilation of the features (see compileFeatures()).
New in version 2.4.
-
compileFeatures
()¶
Compiles the features, thus making the new feature code functionally available in the editor. Equivalent to the “Test” button in the features panel.
New in version 2.5.
-
GSFontMaster
¶
Implementation of the master object. This corresponds with the “Masters” pane in the Font Info. In Glyphs.app, the glyphs of each master are reachable not here, but as layers
attached to the glyphs
attached to the font
object. See the infographic on top for better understanding.
-
class
GSFontMaster
¶ Properties
-
id
¶
Used to identify
Layers
in the Glyphsee
GSGlyph.layers
# ID of first master print(font.masters[0].id) 3B85FBE0-2D2B-4203-8F3D-7112D42D745E # use this master to access the glyph's corresponding layer print(glyph.layers[font.masters[0].id]) <GSLayer "Light" (A)>
Type: unicode -
font
¶
Reference to the
GSFont
object that contains the master. Normally that is set by the app, only if the instance is not actually added to the font, then set this manually.Type: GSFont New in version 2.5.2.
-
name
¶
The human-readable identification of the master, e.g., “Bold Condensed”.
Type: string -
axes
¶
List of floats specifying the positions for each axis
# setting a value for a specific axis master.axes[2] = 12 # setting all values at once master.axes = [100, 12, 3.5]
Type: list New in version 2.5.2.
-
weight
¶
Human-readable weight name, chosen from list in Font Info. For the position in the interpolation design space, use
axes
.Deprecated since version 2.5.2: Use
GSFontMaster.name
instead.Type: string -
width
¶
Human-readable width name, chosen from list in Font Info. For the position in the interpolation design space, use
axes
.Deprecated since version 2.5.2: Use
GSFontMaster.name
instead.Type: string -
customName
¶
The name of the custom interpolation dimension.
Deprecated since version 2.5.2: Use
GSFontMaster.axes
instead.Type: string -
weightValue
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
GSFontMaster.axes
instead.Type: float -
widthValue
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
GSFontMaster.axes
instead.Type: float -
customValue
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
GSFontMaster.axes
instead.Type: float -
customValue1
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
GSFontMaster.axes
instead.Type: float -
customValue2
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
GSFontMaster.axes
instead.Type: float -
customValue3
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
GSFontMaster.axes
instead.Type: float -
ascender
¶
Type: float -
capHeight
¶
Type: float -
xHeight
¶
Type: float -
descender
¶
Type: float -
italicAngle
¶
Type: float -
verticalStems
¶
The vertical stems. This is a list of numbers. For the time being, this can be set only as an entire list at once.
Type: list # Set stems font.masters[0].verticalStems = [10, 11, 20]
-
horizontalStems
¶
The horizontal stems. This is a list of numbers. For the time being, this can be set only as an entire list at once.
Type: list # Set stems font.masters[0].horizontalStems = [10, 11, 20]
-
alignmentZones
¶
Collection of
GSAlignmentZone
objects.Type: list -
blueValues
¶
PS hinting Blue Values calculated from the master’s alignment zones. Read-only.
Type: list -
otherBlues
¶
PS hinting Other Blues calculated from the master’s alignment zones. Read-only.
Type: list -
guides
¶
Collection of
GSGuideLine
objects. These are the font-wide (actually master-wide) red guidelines. For glyph-level guidelines (attached to the layers) seeGSLayer.guides
Type: list -
userData
¶
A dictionary to store user data. Use a unique key, and only use objects that can be stored in a property list (bool, string, list, dict, numbers, NSData), otherwise the data will not be recoverable from the saved file.
Type: dict # set value font.masters[0].userData['rememberToMakeTea'] = True # delete value del font.masters[0].userData['rememberToMakeTea']
-
customParameters
¶
The custom parameters. List of
GSCustomParameter
objects. You can access them by name or by index.# access all parameters for parameter in font.masters[0].customParameters: print(parameter) # set a parameter font.masters[0].customParameters['underlinePosition'] = -135 # delete a parameter del(font.masters[0].customParameters['underlinePosition'])
Type: list, dict -
GSAlignmentZone
¶
Implementation of the alignmentZone object.
There is no distinction between Blue Zones and Other Zones. All negative zones (except the one with position 0) will be exported as Other Zones.
The zone for the baseline should have position 0 (zero) and a negative width.
GSInstance
¶
Implementation of the instance object. This corresponds with the “Instances” pane in the Font Info.
-
class
GSInstance
¶ Properties
Functions
generate()
Properties
-
active
¶
Type: bool -
name
¶
Name of instance. Corresponds to the “Style Name” field in the font info. This is used for naming the exported fonts.
Type: string -
weight
¶
Human-readable weight name, chosen from list in Font Info. For actual position in interpolation design space, use GSInstance.weightValue.
Type: string -
width
¶
Human-readable width name, chosen from list in Font Info. For actual position in interpolation design space, use GSInstance.widthValue.
Type: string -
axes
¶
List of floats specifying the positions for each axis
# setting a value for a specific axis master.axes[2] = 12 # setting all values at once master.axes = [100, 12, 3.5]
Type: list New in version 2.5.2.
-
weightValue
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
axes
instead.Type: float -
widthValue
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
axes
instead.Type: float -
customValue
¶
Value for interpolation in design space.
Deprecated since version 2.5.2: Use
axes
instead.Type: float -
isItalic
¶
Italic flag for style linking
Type: bool -
isBold
¶
Bold flag for style linking
Type: bool -
linkStyle
¶
Linked style
Type: string -
familyName
¶
familyName
Type: string -
preferredFamily
¶
preferredFamily
Type: string -
preferredSubfamilyName
¶
preferredSubfamilyName
Type: string -
windowsFamily
¶
windowsFamily
Type: string -
windowsStyle
¶
windowsStyle This is computed from “isBold” and “isItalic”. Read-only.
Type: string -
windowsLinkedToStyle
¶
windowsLinkedToStyle. Read-only.
Type: string -
fontName
¶
fontName (postscriptFontName)
Type: string -
fullName
¶
fullName (postscriptFullName)
Type: string -
font
¶
Reference to the
GSFont
object that contains the instance. Normally that is set by the app, only if the instance is not actually added to the font, then set this manually.Type: GSFont New in version 2.5.1.
-
customParameters
¶
The custom parameters. List of
GSCustomParameter
objects. You can access them by name or by index.# access all parameters for parameter in font.instances[0].customParameters: print(parameter) # set a parameter font.instances[0].customParameters['hheaLineGap'] = 10 # delete a parameter del(font.instances[0].customParameters['hheaLineGap'])
Type: list, dict -
instanceInterpolations
¶
A dict that contains the interpolation coefficients for each master. This is automatically updated if you change interpolationWeight, interpolationWidth, interpolationCustom. It contains FontMaster IDs as keys and coefficients for that master as values. Or, you can set it manually if you set manualInterpolation to True. There is no UI for this, so you need to do that with a script.
Type: dict -
manualInterpolation
¶
Disables automatic calculation of instanceInterpolations This allowes manual setting of instanceInterpolations.
Type: bool -
interpolatedFontProxy
¶
a proxy font that acts similar to a normal font object but only interpolates the glyphs you ask it for.
It is not properly wrapped yet. So you need to use the ObjectiveC methods directly.
-
interpolatedFont
¶
New in version 2.3.
Returns a ready interpolated
GSFont
object representing this instance. Other than the source object, this interpolated font will contain only one master and one instance.Note: When accessing several properties of such an instance consecutively, it is advisable to create the instance once into a variable and then use that. Otherwise, the instance object will be completely interpolated upon each access. See sample below.
# create instance once interpolated = Glyphs.font.instances[0].interpolatedFont # then access it several times print(interpolated.masters) print(interpolated.instances) (<GSFontMaster "Light" width 100.0 weight 75.0>) (<GSInstance "Web" width 100.0 weight 75.0>)
Type: GSFont
Functions
-
generate
([Format, FontPath, AutoHint, RemoveOverlap, UseSubroutines, UseProductionNames, Containers])¶
Exports the instance. All parameters are optional.
Parameters: - The format of the outlines (str) –
OTF
orTTF
. Default: OTF - FontPath (str) – The destination path for the final fonts. If None, it uses the default location set in the export dialog
- AutoHint (bool) – If autohinting should be applied. Default: True
- RemoveOverlap (bool) – If overlaps should be removed. Default: True
- UseSubroutines (bool) – If to use subroutines for CFF. Default: True
- UseProductionNames (bool) – If to use production names. Default: True
- Containers (bool) – list of container formats. Use any of the following constants:
PLAIN
,WOFF
,WOFF2
,EOT
. Default: PLAIN
Returns: On success, True, on failure error message.
Return type: bool/list
# export all instances as OpenType (.otf) and WOFF2 to user's font folder exportFolder = '/Users/myself/Library/Fonts' for instance in Glyphs.font.instances: instance.generate(FontPath = exportFolder, Containers = [PLAIN, WOFF2]) Glyphs.showNotification('Export fonts', 'The export of %s was successful.' % (Glyphs.font.familyName))
-
lastExportedFilePath
¶
New in version 2.4.2.
Returns a ready interpolated
GSFont
object representing this instance. Other than the source object, this interpolated font will contain only one master and one instance.Note: When accessing several properties of such an instance consecutively, it is advisable to create the instance once into a variable and then use that. Otherwise, the instance object will be completely interpolated upon each access. See sample below.
# create instance once interpolated = Glyphs.font.instances[0].interpolatedFont # then access it several times print(interpolated.masters) print(interpolated.instances) (<GSFontMaster "Light" width 100.0 weight 75.0>) (<GSInstance "Web" width 100.0 weight 75.0>)
Type: unicode -
GSCustomParameter
¶
Implementation of the Custom Parameter object. It stores a name/value pair.
You can append GSCustomParameter objects for example to GSFont.customParameters, but this way you may end up with duplicates. It is best to access the custom parameters through its dictionary interface like this:
# access all parameters
for parameter in font.customParameters:
print(parameter)
# set a parameter
font.customParameters['trademark'] = 'ThisFont is a trademark by MyFoundry.com'
# delete a parameter
del(font.customParameters['trademark'])
GSClass
¶
Implementation of the class object. It is used to store OpenType classes.
For details on how to access them, please look at GSFont.classes
-
class
GSClass
([tag, code])¶ Parameters: - tag – The class name
- code – A list of glyph names, separated by space or newline
name
code
Utilities needed to emulate Python’s interactive interpreter. automatic
active
Properties
-
name
¶
The class name
Type: unicode -
code
¶
A string with space separated glyph names.
Type: unicode -
automatic
¶
Define whether this class should be auto-generated when pressing the ‘Update’ button in the Font Info.
Type: bool -
active
¶
Type: bool New in version 2.5.
GSFeaturePrefix
¶
Implementation of the featurePrefix object. It is used to store things that need to be outside of a feature like standalone lookups.
For details on how to access them, please look at GSFont.featurePrefixes
-
class
GSFeaturePrefix
([tag, code])¶ Parameters: - tag – The Prefix name
- code – The feature code in Adobe FDK syntax
name
code
Utilities needed to emulate Python’s interactive interpreter. automatic
active
Properties
-
name
¶
The FeaturePrefix name
Type: unicode -
code
¶
A String containing feature code.
Type: unicode -
automatic
¶
Define whether this should be auto-generated when pressing the ‘Update’ button in the Font Info.
Type: bool -
active
¶
Type: bool New in version 2.5.
GSFeature
¶
Implementation of the feature object. It is used to implement OpenType Features in the Font Info.
For details on how to access them, please look at GSFont.features
-
class
GSFeature
([tag, code])¶ Parameters: - tag – The feature name
- code – The feature code in Adobe FDK syntax
Properties
name
code
Utilities needed to emulate Python’s interactive interpreter. automatic
notes
active
Functions
update()
Properties
-
name
¶
The feature name
Type: unicode -
code
¶
The Feature code in Adobe FDK syntax.
Type: unicode -
automatic
¶
Define whether this feature should be auto-generated when pressing the ‘Update’ button in the Font Info.
Type: bool -
notes
¶
Some extra text. Is shown in the bottom of the feature window. Contains the stylistic set name parameter
Type: unicode -
active
¶
Type: bool New in version 2.5.
Functions
-
update
()¶
Calls the automatic feature code generator for this feature. You can use this to update all OpenType features before export.
Returns: None # first update all features for feature in font.features: if feature.automatic: feature.update() # then export fonts for instance in font.instances: if instance.active: instance.generate()
GSGlyph
¶
Implementation of the glyph object.
For details on how to access these glyphs, please see GSFont.glyphs
-
class
GSGlyph
([name])¶ Parameters: name – The glyph name Properties
Functions
beginUndo()
endUndo()
updateGlyphInfo()
duplicate()
Properties
-
parent
¶
Reference to the
GSFont
object.Type: GSFont
-
layers
¶
The layers of the glyph, collection of
GSLayer
objects. You can access them either by index or by layer ID, which can be aGSFontMaster.id
. The layer IDs are usually a unique string chosen by Glyphs.app and not set manually. They may look like this: 3B85FBE0-2D2B-4203-8F3D-7112D42D745EType: list, dict # get active layer layer = font.selectedLayers[0] # get glyph of this layer glyph = layer.parent # access all layers of this glyph for layer in glyph.layers: print(layer.name) # access layer of currently selected master of active glyph ... # (also use this to access a specific layer of glyphs selected in the Font View) layer = glyph.layers[font.selectedFontMaster.id] # ... which is exactly the same as: layer = font.selectedLayers[0] # directly access 'Bold' layer of active glyph for master in font.masters: if master.name == 'Bold': id = master.id break layer = glyph.layers[id] # add a new layer newLayer = GSLayer() newLayer.name = '{125, 100}' # (example for glyph-level intermediate master) # you may set the master ID that this layer will be associated with, otherwise the first master will be used newLayer.associatedMasterId = font.masters[-1].id # attach to last master font.glyphs['a'].layers.append(newLayer) # duplicate a layer under a different name newLayer = font.glyphs['a'].layers[0].copy() newLayer.name = 'Copy of layer' # FYI, this will still be the old layer ID (in case of duplicating) at this point print(newLayer.layerId) font.glyphs['a'].layers.append(newLayer) # FYI, the layer will have been assigned a new layer ID by now, after having been appended print(newLayer.layerId) # replace the second master layer with another layer newLayer = GSLayer() newLayer.layerId = font.masters[1].id # Make sure to sync the master layer ID font.glyphs['a'].layers[font.masters[1].id] = newLayer # delete last layer of glyph # (Also works for master layers. They will be emptied) del(font.glyphs['a'].layers[-1]) # delete currently active layer del(font.glyphs['a'].layers[font.selectedLayers[0].layerId])
-
name
¶
The name of the glyph. It will be converted to a “nice name” (afii10017 to A-cy) (you can disable this behavior in font info or the app preference)
Type: unicode -
unicode
¶
String with the hex Unicode value of glyph, if encoded.
Type: unicode -
unicodes
¶
List of String‚ with the hex Unicode values of glyph, if encoded.
Type: unicode -
string
¶
String representation of glyph, if encoded. This is similar to the string representation that you get when copying glyphs into the clipboard.
Type: unicode -
id
¶
An unique identifier for each glyph
Type: string -
category
¶
The category of the glyph. e.g. ‘Letter’, ‘Symbol’ Setting only works if storeCategory is set (see below).
Type: unicode -
storeCategory
¶
Set to True in order to manipulate the category of the glyph (see above). Makes it possible to ship custom glyph data inside a .glyphs file without a separate GlyphData file. Same as Cmd-Alt-i dialog in UI.
Type: bool -
subCategory
¶
The subCategory of the glyph. e.g. ‘Uppercase’, ‘Math’ Setting only works if storeSubCategory is set (see below).
Type: unicode -
storeSubCategory
¶
Set to True in order to manipulate the subCategory of the glyph (see above). Makes it possible to ship custom glyph data inside a .glyphs file without a separate GlyphData file. Same as Cmd-Alt-i dialog in UI.
Type: bool New in version 2.3.
-
script
¶
The script of the glyph, e.g., ‘latin’, ‘arabic’. Setting only works if storeScript is set (see below).
Type: unicode -
storeScript
¶
Set to True in order to manipulate the script of the glyph (see above). Makes it possible to ship custom glyph data inside a .glyphs file without a separate GlyphData file. Same as Cmd-Alt-i dialog in UI.
Type: bool New in version 2.3.
-
productionName
¶
The productionName of the glyph. Setting only works if storeProductionName is set (see below).
Type: unicode New in version 2.3.
-
storeProductionName
¶
Set to True in order to manipulate the productionName of the glyph (see above). Makes it possible to ship custom glyph data inside a .glyphs file without a separate GlyphData file. Same as Cmd-Alt-i dialog in UI.
Type: bool New in version 2.3.
-
glyphInfo
¶
GSGlyphInfo
object for this glyph with detailed information.Type: GSGlyphInfo
-
leftKerningGroup
¶
The leftKerningGroup of the glyph. All glyphs with the same text in the kerning group end up in the same kerning class.
Type: unicode -
rightKerningGroup
¶
The rightKerningGroup of the glyph. All glyphs with the same text in the kerning group end up in the same kerning class.
Type: unicode -
leftKerningKey
¶
The key to be used with the kerning functions (
GSFont.kerningForPair()
,GSFont.setKerningForPair()
GSFont.removeKerningForPair()
).If the glyph has a :att:`leftKerningGroup <GSGlyph.leftKerningGroup>` attribute, the internally used @MMK_R_xx notation will be returned (note that the R in there stands for the right side of the kerning pair for LTR fonts, which corresponds to the left kerning group of the glyph). If no group is given, the glyph’s name will be returned.
Type: string # Set kerning for 'T' and all members of kerning class 'a' # For LTR fonts, always use the .rightKerningKey for the first (left) glyph of the pair, .leftKerningKey for the second (right) glyph. font.setKerningForPair(font.selectedFontMaster.id, font.glyphs['T'].rightKerningKey, font.glyphs['a'].leftKerningKey, -60) # which corresponds to: font.setKerningForPair(font.selectedFontMaster.id, 'T', '@MMK_R_a', -60)
New in version 2.4.
-
rightKerningKey
¶
The key to be used with the kerning functions (
GSFont.kerningForPair()
,GSFont.setKerningForPair()
GSFont.removeKerningForPair()
).If the glyph has a :att:`rightKerningGroup <GSGlyph.rightKerningGroup>` attribute, the internally used @MMK_L_xx notation will be returned (note that the L in there stands for the left side of the kerning pair for LTR fonts, which corresponds to the right kerning group of the glyph). If no group is given, the glyph’s name will be returned.
See above for an example.
Type: string New in version 2.4.
-
leftMetricsKey
¶
The leftMetricsKey of the glyph. This is a reference to another glyph by name or formula. It is used to synchronize the metrics with the linked glyph.
Type: unicode -
rightMetricsKey
¶
The rightMetricsKey of the glyph. This is a reference to another glyph by name or formula. It is used to synchronize the metrics with the linked glyph.
Type: unicode -
widthMetricsKey
¶
The widthMetricsKey of the glyph. This is a reference to another glyph by name or formula. It is used to synchronize the metrics with the linked glyph.
Type: unicode -
export
¶
Defines whether glyph will export upon font generation
Type: bool -
color
¶
Color marking of glyph in UI
Type: int glyph.color = 0 # red glyph.color = 1 # orange glyph.color = 2 # brown glyph.color = 3 # yellow glyph.color = 4 # light green glyph.color = 5 # dark green glyph.color = 6 # light blue glyph.color = 7 # dark blue glyph.color = 8 # purple glyph.color = 9 # magenta glyph.color = 10 # light gray glyph.color = 11 # charcoal glyph.color = 9223372036854775807 # not colored, white
-
colorObject
¶
NSColor object of glyph color, useful for drawing in plugins.
Type: NSColor # use glyph color to draw the outline glyph.colorObject.set() # Get RGB (and alpha) values (as float numbers 0..1, multiply with 256 if necessary) R, G, B, A = glyph.colorObject.colorUsingColorSpace_(NSColorSpace.genericRGBColorSpace()).getRed_green_blue_alpha_(None, None, None, None) print(R, G, B) 0.617805719376 0.958198726177 0.309286683798 print(round(R * 256), int(G * 256), int(B * 256)) 158 245 245 # Draw layer glyph.layers[0].bezierPath.fill() # set the glyph color. glyph.colorObject = NSColor.colorWithDeviceRed_green_blue_alpha_(247.0 / 255.0, 74.0 / 255.0, 62.9 / 255.0, 1) new in 2.4.2: glyph.colorObject = (247.0, 74.0, 62.9) # or glyph.colorObject = (247.0, 74.0, 62.9, 1) # or glyph.colorObject = (0.968, 0.29, 0.247, 1) #
New in version 2.3.
-
note
¶
Type: unicode -
selected
¶
Return True if the Glyph is selected in the Font View. This is different to the property font.selectedLayers which returns the selection from the active tab.
Type: bool # access all selected glyphs in the Font View for glyph in font.glyphs: if glyph.selected: print(glyph)
-
mastersCompatible
¶
New in version 2.3.
Return True when all layers in this glyph are compatible (same components, anchors, paths etc.)
Type: bool -
userData
¶
New in version 2.3.
A dictionary to store user data. Use a unique key and only use objects that can be stored in a property list (string, list, dict, numbers, NSData) otherwise the data will not be recoverable from the saved file.
Type: dict # set value glyph.userData['rememberToMakeCoffee'] = True # delete value del glyph.userData['rememberToMakeCoffee']
-
smartComponentAxes
¶
New in version 2.3.
A list of
GSSmartComponentAxis
objects.These are the axis definitions for the interpolations that take place within the Smart Components. Corresponds to the ‘Properties’ tab of the glyph’s ‘Show Smart Glyph Settings’ dialog.
Also see https://glyphsapp.com/tutorials/smart-components for reference.
Type: list # Adding two interpolation axes to the glyph axis1 = GSSmartComponentAxis() axis1.name = 'crotchDepth' axis1.topValue = 0 axis1.bottomValue = -100 g.smartComponentAxes.append(axis1) axis2 = GSSmartComponentAxis() axis2.name = 'shoulderWidth' axis2.topValue = 100 axis2.bottomValue = 0 g.smartComponentAxes.append(axis2) # Deleting one axis del g.smartComponentAxes[1]
-
lastChange
¶
New in version 2.3.
Change date when glyph was last changed as datetime.
Check Python’s
time
module for how to use the timestamp.Functions
-
beginUndo
()¶
Call this before you do a longer running change to the glyph. Be extra careful to call Glyph.endUndo() when you are finished.
-
endUndo
()¶
This closes a undo group that was opened by a previous call of Glyph.beginUndo(). Make sure that you call this for each beginUndo() call.
-
updateGlyphInfo
(changeName = True)¶
Updates all information like name, unicode etc. for this glyph.
-
duplicate
([name])¶
Duplicate the glyph under a new name and return it.
If no name is given, .00n will be appended to it.
-
GSLayer
¶
Implementation of the layer object.
For details on how to access these layers, please see GSGlyph.layers
-
class
GSLayer
¶ Properties
Functions
-
parent
¶
Reference to the
glyph
object that this layer is attached to.Type: GSGlyph
-
name
¶
Name of layer
Type: unicode -
master
¶
Master that this layer is connected to. Read only.
Type: GSFontMaster -
associatedMasterId
¶
The ID of the
fontMaster
this layer belongs to, in case this isn’t a master layer. Every layer that isn’t a master layer needs to be attached to one master layer.Type: unicode # add a new layer newLayer = GSLayer() newLayer.name = '{125, 100}' # (example for glyph-level intermediate master) # you may set the master ID that this layer will be associated with, otherwise the first master will be used newLayer.associatedMasterId = font.masters[-1].id # attach to last master font.glyphs['a'].layers.append(newLayer)
-
layerId
¶
The unique layer ID is used to access the layer in the
glyphs
layer dictionary.For master layers this should be the id of the
fontMaster
. It could look like this: “FBCA074D-FCF3-427E-A700-7E318A949AE5”Type: unicode # see ID of active layer id = font.selectedLayers[0].layerId print(id) FBCA074D-FCF3-427E-A700-7E318A949AE5 # access a layer by this ID layer = font.glyphs['a'].layers[id] layer = font.glyphs['a'].layers['FBCA074D-FCF3-427E-A700-7E318A949AE5'] # for master layers, use ID of masters layer = font.glyphs['a'].layers[font.masters[0].id]
-
color
¶
Color marking of glyph in UI
Type: int glyph.color = 0 # red glyph.color = 1 # orange glyph.color = 2 # brown glyph.color = 3 # yellow glyph.color = 4 # light green glyph.color = 5 # dark green glyph.color = 6 # light blue glyph.color = 7 # dark blue glyph.color = 8 # purple glyph.color = 9 # magenta glyph.color = 10 # light gray glyph.color = 11 # charcoal glyph.color = 9223372036854775807 # not colored, white
-
colorObject
¶
New in version 2.3.
NSColor object of layer color, useful for drawing in plugins.
Type: NSColor # use layer color to draw the outline layer.colorObject.set() # Get RGB (and alpha) values (as float numbers 0..1, multiply with 256 if necessary) R, G, B, A = layer.colorObject.colorUsingColorSpace_(NSColorSpace.genericRGBColorSpace()).getRed_green_blue_alpha_(None, None, None, None) print(R, G, B) 0.617805719376 0.958198726177 0.309286683798 print(round(R * 256), int(G * 256), int(B * 256)) 158 245 245 # Draw layer layer.bezierPath.fill() # set the layer color. layer.colorObject = NSColor.colorWithDeviceRed_green_blue_alpha_(247.0 / 255.0, 74.0 / 255.0, 62.9 / 255.0, 1)
-
components
¶
Collection of
GSComponent
objectsType: list layer = Glyphs.font.selectedLayers[0] # current layer # add component layer.components.append(GSComponent('dieresis')) # add component at specific position layer.components.append(GSComponent('dieresis', NSPoint(100, 100))) # delete specific component for i, component in enumerate(layer.components): if component.componentName == 'dieresis': del(layer.components[i]) break # copy components from another layer import copy layer.components = copy.copy(anotherlayer.components) # copy one component to another layer layer.components.append(anotherlayer.component[0].copy())
-
guides
¶
List of
GSGuideLine
objects.Type: list layer = Glyphs.font.selectedLayers[0] # current layer # access all guides for guide in layer.guides: print(guide) # add guideline newGuide = GSGuideLine() newGuide.position = NSPoint(100, 100) newGuide.angle = -10.0 layer.guides.append(newGuide) # delete guide del(layer.guides[0]) # copy guides from another layer import copy layer.guides = copy.copy(anotherlayer.guides)
-
annotations
¶
List of
GSAnnotation
objects.Type: list layer = Glyphs.font.selectedLayers[0] # current layer # access all annotations for annotation in layer.annotations: print(annotation) # add new annotation newAnnotation = GSAnnotation() newAnnotation.type = TEXT newAnnotation.text = 'Fuck, this curve is ugly!' layer.annotations.append(newAnnotation) # delete annotation del(layer.annotations[0]) # copy annotations from another layer import copy layer.annotations = copy.copy(anotherlayer.annotations)
-
hints
¶
List of
GSHint
objects.Type: list layer = Glyphs.font.selectedLayers[0] # current layer # access all hints for hint in layer.hints: print(hint) # add a new hint newHint = GSHint() # change behaviour of hint here, like its attachment nodes layer.hints.append(newHint) # delete hint del(layer.hints[0]) # copy hints from another layer import copy layer.hints = copy.copy(anotherlayer.hints) # remember to reconnect the hints' nodes with the new layer's nodes
-
anchors
¶
List of
GSAnchor
objects.Type: list, dict layer = Glyphs.font.selectedLayers[0] # current layer # access all anchors: for a in layer.anchors: print(a) # add a new anchor layer.anchors['top'] = GSAnchor() # delete anchor del(layer.anchors['top']) # copy anchors from another layer import copy layer.anchors = copy.copy(anotherlayer.anchors)
-
paths
¶
List of
GSPath
objects.Type: list # access all paths for path in layer.paths: print(path) # delete path del(layer.paths[0]) # copy paths from another layer import copy layer.paths = copy.copy(anotherlayer.paths)
-
selection
List of all selected objects in the glyph. Read-only.
This list contains all selected items, including nodes, anchors, guidelines etc. If you want to work specifically with nodes, for instance, you may want to cycle through the nodes (or anchors etc.) and check whether they are selected. See example below.
# access all selected nodes for path in layer.paths: for node in path.nodes: # (or path.anchors etc.) print(node.selected) # clear selection layer.clearSelection()
Type: list -
LSB
¶
Left sidebearing
Type: float -
RSB
¶
Right sidebearing
Type: float -
TSB
¶
Top sidebearing
Type: float -
BSB
¶
Bottom sidebearing
Type: float -
width
¶
Glyph width
Type: float -
leftMetricsKey
¶
The leftMetricsKey of the layer. This is a reference to another glyph by name or formula. It is used to synchronize the metrics with the linked glyph.
Type: unicode -
rightMetricsKey
¶
The rightMetricsKey of the layer. This is a reference to another glyph by name or formula. It is used to synchronize the metrics with the linked glyph.
Type: unicode -
widthMetricsKey
¶
The widthMetricsKey of the layer. This is a reference to another glyph by name or formula. It is used to synchronize the metrics with the linked glyph.
Type: unicode -
bounds
¶
Bounding box of whole glyph as NSRect. Read-only.
Type: NSRect layer = Glyphs.font.selectedLayers[0] # current layer # origin print(layer.bounds.origin.x, layer.bounds.origin.y) # size print(layer.bounds.size.width, layer.bounds.size.height)
-
selectionBounds
¶
Bounding box of the layer’s selection (nodes, anchors, components etc). Read-only.
Type: NSRect -
background
¶
The background layer
Type: GSLayer
-
backgroundImage
¶
The background image. It will be scaled so that 1 em unit equals 1 of the image’s pixels.
Type: GSBackgroundImage
# set background image layer.backgroundImage = GSBackgroundImage('/path/to/file.jpg') # remove background image layer.backgroundImage = None
-
bezierPath
¶
New in version 2.3.
The layer as an NSBezierPath object. Useful for drawing glyphs in plug-ins.
# draw the path into the Edit view NSColor.redColor().set() layer.bezierPath.fill()
Type: NSBezierPath -
openBezierPath
¶
New in version 2.3.
All open paths of the layer as an NSBezierPath object. Useful for drawing glyphs as outlines in plug-ins.
# draw the path into the Edit view NSColor.redColor().set() layer.openBezierPath.stroke()
Type: NSBezierPath -
completeBezierPath
¶
New in version 2.3.1.
The layer as an NSBezierPath object including paths from components. Useful for drawing glyphs in plug-ins.
# draw the path into the Edit view NSColor.redColor().set() layer.completeBezierPath.fill()
Type: NSBezierPath -
completeOpenBezierPath
¶
New in version 2.3.1.
All open paths of the layer as an NSBezierPath object including paths from components. Useful for drawing glyphs as outlines in plugins.
# draw the path into the Edit view NSColor.redColor().set() layer.completeOpenBezierPath.stroke()
Type: NSBezierPath -
isAligned
¶
New in version 2.3.1.
Indicates if the components are auto aligned.
Type: bool -
isSpecialLayer
¶
If the layer is a brace, bracket or a smart component layer
Type: bool -
userData
¶
New in version 2.3.
A dictionary to store user data. Use a unique key and only use objects that can be stored in a property list (string, list, dict, numbers, NSData) otherwise the data will not be recoverable from the saved file.
Type: dict # set value layer.userData['rememberToMakeCoffee'] = True # delete value del layer.userData['rememberToMakeCoffee']
-
smartComponentPoleMapping
¶
New in version 2.3.
Maps this layer to the poles on the interpolation axes of the Smart Glyph. The dictionary keys are the names of the
GSSmartComponentAxis
objects. The values are 1 for bottom pole and 2 for top pole. Corresponds to the ‘Layers’ tab of the glyph’s ‘Show Smart Glyph Settings’ dialog.Also see https://glyphsapp.com/tutorials/smart-components for reference.
Type: dict, int # Map layers to top and bottom poles: crotchDepthAxis = glyph.smartComponentAxes['crotchDepth'] shoulderWidthAxis = glyph.smartComponentAxes['shoulderWidth'] for layer in glyph.layers: # Regular layer if layer.name == 'Regular': layer.smartComponentPoleMapping[crotchDepthAxis.id] = 2 layer.smartComponentPoleMapping[shoulderWidthAxis.id] = 2 # NarrowShoulder layer elif layer.name == 'NarrowShoulder': layer.smartComponentPoleMapping[crotchDepthAxis.id] = 2 layer.smartComponentPoleMapping[shoulderWidthAxis.id] = 1 # LowCrotch layer elif layer.name == 'LowCrotch': layer.smartComponentPoleMapping[crotchDepthAxis.id] = 1 layer.smartComponentPoleMapping[shoulderWidthAxis.id] = 2
Functions
-
decomposeComponents
()¶ Decomposes all components of the layer at once.
-
decomposeCorners
()¶ New in version 2.4.
Decomposes all corners of the layer at once.
-
compareString
()¶ Returns a string representing the outline structure of the glyph, for compatibility comparison.
Returns: The comparison string Return type: string layer = Glyphs.font.selectedLayers[0] # current layer print(layer.compareString()) oocoocoocoocooc_oocoocoocloocoocoocoocoocoocoocoocooc_
-
connectAllOpenPaths
()¶ Closes all open paths when end points are further than 1 unit away from each other.
-
copyDecomposedLayer
()¶ Returns a copy of the layer with all components decomposed.
Returns: A new layer object Return type: GSLayer
-
syncMetrics
()¶ Take over LSB and RSB from linked glyph.
# sync metrics of all layers of this glyph for layer in glyph.layers: layer.syncMetrics()
-
correctPathDirection
()¶ Corrects the path direction.
-
removeOverlap
()¶ Joins all contours.
Parameters: checkSelection – if the selection will be considered. Default: False
-
roundCoordinates
()¶ New in version 2.3.
Round the positions of all coordinates to the grid (size of which is set in the Font Info).
-
addNodesAtExtremes
()¶ New in version 2.3.
Add nodes at layer’s extrema, e.g., top, bottom etc.
-
applyTransform
()¶
Apply a transformation matrix to the layer.
layer = Glyphs.font.selectedLayers[0] # current layer layer.applyTransform([ 0.5, # x scale factor 0.0, # x skew factor 0.0, # y skew factor 0.5, # y scale factor 0.0, # x position 0.0 # y position ])
-
beginChanges
()¶ Call this before you do bigger changes to the Layer. This will increase performance and prevent undo problems. Always call layer.endChanges() if you are finished.
-
endChanges
()¶ Call this if you have called layer.beginChanges before. Make sure to group bot calls properly.
-
cutBetweenPoints
(Point1, Point2)¶ Cuts all paths that intersect the line from Point1 to Point2
Parameters: - Point1 – one point
- Point2 – the other point
layer = Glyphs.font.selectedLayers[0] # current layer # cut glyph in half horizontally at y=100 layer.cutBetweenPoints(NSPoint(0, 100), NSPoint(layer.width, 100))
-
intersectionsBetweenPoints
(Point1, Point2, components = False)¶ Return all intersection points between a measurement line and the paths in the layer. This is basically identical to the measurement tool in the UI.
Normally, the first returned point is the starting point, the last returned point is the end point. Thus, the second point is the first intersection, the second last point is the last intersection.
Parameters: - Point1 – one point
- Point2 – the other point
- components – if components should be measured. Default: False
layer = Glyphs.font.selectedLayers[0] # current layer # show all intersections with glyph at y=100 intersections = layer.intersectionsBetweenPoints((-1000, 100), (layer.width+1000, 100)) print(intersections) # left sidebearing at measurement line print(intersections[1].x) # right sidebearing at measurement line print(layer.width - intersections[-2].x)
-
addMissingAnchors
()¶ Adds missing anchors defined in the glyph database.
-
clearSelection
()¶ New in version 2.3.
Unselect all selected items in this layer.
-
clear
()¶ New in version 2.3.
Remove all elements from layer.
-
swapForegroundWithBackground
()¶ New in version 2.3.
Swap Foreground layer with Background layer.
-
reinterpolate
()¶ New in version 2.3.
Re-interpolate a layer according the other layers and its interpolation values.
Applies to both master layers as well as brace layers and is equivalent to the ‘Re-Interpolate’ command from the Layers palette.
-
GSAnchor
¶
Implementation of the anchor object.
For details on how to access them, please see GSLayer.anchors
-
class
GSAnchor
([name, pt])¶ Parameters: - name – the name of the anchor
- pt – the position of the anchor
Properties
position
name
selected
Properties
-
position
¶
The position of the anchor
Type: NSPoint # read position print(layer.anchors['top'].position.x, layer.anchors['top'].position.y) # set position layer.anchors['top'].position = NSPoint(175, 575) # increase vertical position by 50 units layer.anchors['top'].position = NSPoint(layer.anchors['top'].position.x, layer.anchors['top'].position.y + 50)
-
name
¶
The name of the anchor
Type: unicode -
selected
¶
Selection state of anchor in UI.
# select anchor layer.anchors[0].selected = True # log selection state print(layer.anchors[0].selected)
Type: bool
GSComponent
¶
Implementation of the component object.
For details on how to access them, please see GSLayer.components
-
class
GSComponent
(glyph[, position])¶ Parameters: - glyph – a
GSGlyph
object or the glyph name - position – the position of the component as NSPoint
Properties
position
scale
rotation
componentName
component
layer
transform
bounds
automaticAlignment
anchor
selected
smartComponentValues
bezierPath
userData
Functions
decompose()
applyTransform()
Properties
-
position
¶
The Position of the component.
Type: NSPoint -
scale
¶
Scale factor of image.
A scale factor of 1.0 (100%) means that 1 em unit equals 1 of the image’s pixels.
This sets the scale factor for x and y scale simultaneously. For separate scale factors, please use the transformation matrix.
Type: float or tuple -
rotation
¶
Rotation angle of component.
Type: float -
componentName
¶
The glyph name the component is pointing to.
Type: unicode -
name
¶
The glyph name the component is pointing to.
Type: unicode New in version 2.5.
-
component
¶
The
GSGlyph
the component is pointing to. This is read-only. In order to change the referenced base glyph, setcomponentName
to the new glyph name.Type: GSGlyph
-
componentLayer
¶
The
GSLayer
the component is pointing to. This is read-only. In order to change the referenced base glyph, setcomponentName
to the new glyph name.For Smart Components, the componentLayer contains the interpolated result.
Type: GSLayer
New in version 2.5.
-
transform
¶
Transformation matrix of the component.
component = layer.components[0] component.transform = (( 0.5, # x scale factor 0.0, # x skew factor 0.0, # y skew factor 0.5, # y scale factor 0.0, # x position 0.0 # y position ))
Type: NSAffineTransformStruct -
bounds
¶
Bounding box of the component, read-only
Type: NSRect component = layer.components[0] # first component # origin print(component.bounds.origin.x, component.bounds.origin.y) # size print(component.bounds.size.width, component.bounds.size.height)
-
automaticAlignment
¶
Defines whether the component is automatically aligned.
Type: bool -
alignment
¶
New in version 2.5.
TODO
-
locked
¶
New in version 2.5.
If the component is locked TODO
Type: bool -
anchor
¶
If more than one anchor/_anchor pair would match, this property can be used to set the anchor to use for automatic alignment
This can be set from the anchor button in the component info box in the UI
Type: unicode -
selected
¶
Selection state of component in UI.
# select component layer.components[0].selected = True # print(selection state) print(layer.components[0].selected)
Type: bool -
smartComponentValues
¶
New in version 2.3.
Dictionary of interpolations values of the Smart Component. Key are the names, values are between the top and the bottom value of the corresponding
GSSmartComponentAxis
objects. Corresponds to the values of the ‘Smart Component Settings’ dialog. Returns None if the component is not a Smart Component.Also see https://glyphsapp.com/tutorials/smart-components for reference.
Type: dict, int # Narrow shoulders of m glyph = font.glyphs['m'] glyph.layers[0].components[1].smartComponentValues['shoulderWidth'] = 30 # First shoulder. Index is 1, given that the stem is also a component with index 0 glyph.layers[0].components[2].smartComponentValues['shoulderWidth'] = 30 # Second shoulder. Index is 2, given that the stem is also a component with index 0 # Low crotch of h glyph = font.glyphs['h'] crotchDepthAxis = glyph.smartComponentAxes['crotchDepth'] glyph.layers[0].components[1].smartComponentValues[crotchDepthAxis.id] = -77 # Shoulder. Index is 1, given that the stem is also a component with index 0 # Check whether a component is a smart component for component in layer.components: if component.smartComponentValues is not None: # do stuff
-
bezierPath
¶
New in version 2.3.
The component as an NSBezierPath object. Useful for drawing glyphs in plugins.
# draw the path into the Edit view NSColor.redColor().set() layer.components[0].bezierPath.fill()
Type: NSBezierPath -
userData
¶
New in version 2.5.
A dictionary to store user data. Use a unique key and only use objects that can be stored in a property list (string, list, dict, numbers, NSData) otherwise the data will not be recoverable from the saved file.
Type: dict # set value component.userData['rememberToMakeCoffee'] = True # delete value del component.userData['rememberToMakeCoffee']
Functions
-
decompose
(doAnchors = True, doHints = True)¶
Parameters: - doAnchors – get anchors from components
- doHints – get hints from components
Decomposes the component.
-
applyTransform
()¶
Apply a transformation matrix to the component.
component = layer.components[0] component.applyTransform(( 0.5, # x scale factor 0.0, # x skew factor 0.0, # y skew factor 0.5, # y scale factor 0.0, # x position 0.0 # y position ))
- glyph – a
GSSmartComponentAxis
¶
Implementation of the Smart Component interpolation axis object.
For details on how to access them, please see GSGlyph.smartComponentAxes
New in version 2.3.
-
class
GSSmartComponentAxis
¶ Properties
name
topValue
bottomValue
Properties
-
name
¶
Name of the axis. The name is for display purpose only.
Type: str -
id
¶
Id of the axis. This Id will be used to map the Smart Glyph’s layers to the poles of the interpolation. See
GSLayer.smartComponentPoleMapping
Type: str New in version 2.5.
-
topValue
¶
Top end (pole) value on interpolation axis.
Type: int, float -
bottomValue
¶
Bottom end (pole) value on interpolation axis.
Type: int, float -
GSPath
¶
Implementation of the path object.
For details on how to access them, please see GSLayer.paths
If you build a path in code, make sure that the structure is valid. A curve node has to be preceded by two off-curve nodes. And an open path has to start with a line node.
-
class
GSPath
¶ Properties
parent
nodes
segments
closed
direction
bounds
selected
bezierPath
Functions
reverse()
addNodesAtExtremes()
applyTransform()
Properties
-
parent
¶
Reference to the
layer
object.Type: GSLayer
-
nodes
¶
A list of
GSNode
objectsType: list # access all nodes for path in layer.paths: for node in path.nodes: print(node)
-
segments
¶
A list of segments as NSPoint objects. Two objects represent a line, four represent a curve. Start point of the segment is included.
Type: list # access all segments for path in layer.paths: for segment in path.segments: print(segment)
-
closed
¶
Returns True if the the path is closed
Type: bool -
direction
¶
Path direction. -1 for counter clockwise, 1 for clockwise.
Type: int -
bounds
¶
Bounding box of the path, read-only
Type: NSRect path = layer.paths[0] # first path # origin print(path.bounds.origin.x, path.bounds.origin.y) # size print(path.bounds.size.width, path.bounds.size.height)
-
selected
¶
Selection state of path in UI.
# select path layer.paths[0].selected = True # print(selection state) print(layer.paths[0].selected)
Type: bool -
bezierPath
¶
New in version 2.3.
The same path as an NSBezierPath object. Useful for drawing glyphs in plugins.
# draw the path into the Edit view NSColor.redColor().set() layer.paths[0].bezierPath.fill()
Type: NSBezierPath Functions
-
reverse
()¶ Reverses the path direction
-
draw the object with a fontTools pen
addNodesAtExtremes
()¶Add nodes at path’s extrema, e.g., top, bottom etc.
New in version 2.3.
applyTransform
()¶Apply a transformation matrix to the path.
path = layer.paths[0] path.applyTransform(( 0.5, # x scale factor 0.0, # x skew factor 0.0, # y skew factor 0.5, # y scale factor 0.0, # x position 0.0 # y position ))
GSNode
¶
Implementation of the node object.
For details on how to access them, please see GSPath.nodes
-
class
GSNode
([pt, type = type])¶ Parameters: - pt – The position of the node.
- type – The type of the node, LINE, CURVE or OFFCURVE
Properties
position
type
connection
selected
index
nextNode
prevNode
name
Functions
makeNodeFirst()
toggleConnection()
Properties
-
position
¶
The position of the node.
Type: NSPoint -
type
¶
The type of the node, LINE, CURVE or OFFCURVE
Always compare against the constants, never against the actual value.
Type: str -
smooth
¶
If it is a smooth connection or not
Type: BOOL New in version 2.3.
-
connection
¶
The type of the connection, SHARP or SMOOTH
Type: string Deprecated since version 2.3: Use
smooth
instead.-
selected
¶
Selection state of node in UI.
# select node layer.paths[0].nodes[0].selected = True # print(selection state) print(layer.paths[0].nodes[0].selected)
Type: bool -
index
¶
Returns the index of the node in the containing path or maxint if it is not in a path.
Type: int New in version 2.3.
-
nextNode
¶
Returns the next node in the path.
Please note that this is regardless of the position of the node in the path and will jump across the path border to the beginning of the path if the current node is the last.
If you need to take into consideration the position of the node in the path, use the node’s index attribute and check it against the path length.
print(layer.paths[0].nodes[0].nextNode # returns the second node in the path (index 0 + 1)) print(layer.paths[0].nodes[-1].nextNode # returns the first node in the path (last node >> jumps to beginning of path)) # check if node is last node in path (with at least two nodes) print(layer.paths[0].nodes[0].index == (len(layer.paths[0].nodes) - 1)) # returns False for first node print(layer.paths[0].nodes[-1].index == (len(layer.paths[0].nodes) - 1)) # returns True for last node
Type: GSNode New in version 2.3.
-
prevNode
¶
Returns the previous node in the path.
Please note that this is regardless of the position of the node in the path, and will jump across the path border to the end of the path if the current node is the first.
If you need to take into consideration the position of the node in the path, use the node’s index attribute and check it against the path length.
print(layer.paths[0].nodes[0].prevNode) # returns the last node in the path (first node >> jumps to end of path) print(layer.paths[0].nodes[-1].prevNode) # returns second last node in the path # check if node is first node in path (with at least two nodes) print(layer.paths[0].nodes[0].index == 0) # returns True for first node print(layer.paths[0].nodes[-1].index == 0) # returns False for last node
Type: GSNode New in version 2.3.
-
name
¶
Attaches a name to a node.
Type: unicode New in version 2.3.
-
userData
¶
A dictionary to store user data. Use a unique key and only use objects that can be stored in a property list (string, list, dict, numbers, NSData) otherwise the data will not be recoverable from the saved file.
Type: dict # set value node.userData['rememberToMakeCoffee'] = True # delete value del node.userData['rememberToMakeCoffee']
New in version 2.4.1.
Functions
-
makeNodeFirst
()¶ Turn this node into the start point of the path.
-
toggleConnection
()¶ Toggle between sharp and smooth connections.
GSGuideLine
¶
Implementation of the guide object.
For details on how to access them, please see GSLayer.guides
-
class
GSGuideLine
¶ Properties
position
angle
name
selected
-
position
¶
The position of the node.
Type: NSPoint -
angle
¶
Angle
Type: float -
name
¶
a optional name
Type: unicode -
selected
¶
Selection state of guideline in UI.
# select guideline layer.guidelines[0].selected = True # print(selection state) print(layer.guidelines[0].selected)
Type: bool -
GSAnnotation
¶
Implementation of the annotation object.
For details on how to access them, please see GSLayer.annotations
-
class
GSAnnotation
¶ position
type
text
angle
width
Properties
-
position
¶
The position of the annotation.
Type: NSPoint -
type
¶
The type of the annotation.
Available constants are:
TEXT
ARROW
CIRCLE
PLUS
MINUS
Type: int -
text
¶
The content of the annotation. Only useful if type == TEXT
Type: unicode -
angle
¶
The angle of the annotation.
Type: float -
width
¶
The width of the annotation.
Type: float -
GSHint
¶
Implementation of the hint object.
For details on how to access them, please see GSLayer.hints
-
class
GSHint
¶ parent
originNode
targetNode
otherNode1
otherNode2
type
horizontal
selected
Properties
-
parent
¶
Parent layer of hint.
Type: GSLayer New in version 2.4.2.
-
originNode
¶
The first node the hint is attached to.
Type: GSNode
-
targetNode
¶
The the second node this hint is attached to. In the case of a ghost hint, this value will be empty.
Type: GSNode
-
otherNode1
¶
A third node this hint is attached to. Used for Interpolation or Diagonal hints.
Type: GSNode
-
otherNode2
¶
A fourth node this hint is attached to. Used for Diagonal hints.
Type: GSNode
-
type
¶
See Constants section at the bottom of the page.
Type: int -
options
¶
Stores extra options for the hint. For TT hints, that might be the rounding settings. See Constants section at the bottom of the page.
Type: int -
horizontal
¶
True if hint is horizontal, False if vertical.
Type: bool -
selected
¶
Selection state of hint in UI.
# select hint layer.hints[0].selected = True # print(selection state) print(layer.hints[0].selected)
Type: bool -
name
¶
New in version 2.3.1.
Name of the hint. This is the referenced glyph for corner and cap components.
Type: string -
stem
¶
New in version 2.4.2.
Index of TrueType stem that this hint is attached to. The stems are defined in the custom parameter “TTFStems” per master.
For no stem, value is -1.
For automatic, value is -2.
Type: integer -
GSBackgroundImage
¶
Implementation of background image.
For details on how to access it, please see GSLayer.backgroundImage
-
class
GSBackgroundImage
([path])¶ Parameters: path – Initialize with an image file (optional) Properties
path
image
crop
locked
position
scale
rotation
transform
alpha
Functions
resetCrop()
scaleWidthToEmUnits()
scaleHeightToEmUnits()
Properties
-
path
¶
Path to image file.
Type: unicode -
image
¶
NSImage
object of background image, read-only (as in: not settable)Type: NSImage
-
crop
¶
Crop rectangle. This is relative to the image size in pixels, not the font’s em units (just in case the image is scaled to something other than 100%).
Type: NSRect
# change cropping layer.backgroundImage.crop = NSRect(NSPoint(0, 0), NSPoint(1200, 1200))
-
locked
¶
Defines whether image is locked for access in UI.
Type: bool -
alpha
¶
Defines the transparence of the image in the Edit view. Default is 50%, possible values are 10–100.
To reset it to default, set it to anything other than the allowed values.
Type: int New in version 2.3.
-
position
¶
Position of image in font units.
Type: NSPoint
# change position layer.backgroundImage.position = NSPoint(50, 50)
-
scale
¶
Scale factor of image.
A scale factor of 1.0 (100%) means that 1 font unit is equal to 1 point.
Set the scale factor for x and y scale simultaneously with an integer or a float value. For separate scale factors, please use a tuple.
# change scale layer.backgroundImage.scale = 1.2 # changes x and y to 120% layer.backgroundImage.scale = (1.1, 1.2) # changes x to 110% and y to 120%
Type: tuple -
rotation
¶
Rotation angle of image.
Type: float -
transform
¶
Transformation matrix.
Type: NSAffineTransformStruct
# change transformation layer.backgroundImage.transform = (( 1.0, # x scale factor 0.0, # x skew factor 0.0, # y skew factor 1.0, # y scale factor 0.0, # x position 0.0 # y position ))
Functions
-
resetCrop
()¶
Resets the cropping to the image’s original dimensions.
-
scaleWidthToEmUnits
()¶
Scale the image’s cropped width to a certain em unit value, retaining its aspect ratio.
# fit image in layer's width layer.backgroundImage.scaleWidthToEmUnits(layer.width)
-
scaleHeightToEmUnits
()¶
Scale the image’s cropped height to a certain em unit value, retaining its aspect ratio.
# position image's origin at descender line layer.backgroundImage.position = NSPoint(0, font.masters[0].descender) # scale image to UPM value layer.backgroundImage.scaleHeightToEmUnits(font.upm)
-
GSEditViewController
¶
Implementation of the GSEditViewController object, which represents Edit tabs in the UI.
For details on how to access them, please look at GSFont.tabs
-
class
GSEditViewController
¶ Properties
parent
text
layers
composedLayers
scale
viewPort
bounds
selectedLayerOrigin
textCursor
textRange
direction
features
previewInstances
previewHeight
bottomToolbarHeight
Functions
close()
saveToPDF()
Properties
-
parent
¶
The
GSFont
object that this tab belongs to.Type: GSFont
-
text
¶
The text of the tab, either as text, or slash-escaped glyph names, or mixed. OpenType features will be applied after the text has been changed.
Type: Unicode -
layers
¶
Alternatively, you can set (and read) a list of
GSLayer
objects. These can be any of the layers of a glyph. OpenType features will be applied after the layers have been changed.Type: list font.tabs[0].layers = [] # display all layers of one glyph next to each other for layer in font.glyphs['a'].layers: font.tabs[0].layers.append(layer) # append line break font.tabs[0].layers.append(GSControlLayer(10)) # 10 being the ASCII code of the new line character (\n)
-
composedLayers
¶
Similar to the above, but this list contains the
GSLayer
objects after the OpenType features have been applied (seeGSEditViewController.features
). Read-only.Type: list New in version 2.4.
-
scale
¶
Scale (zoom factor) of the Edit view. Useful for drawing activity in plugins.
The scale changes with every zoom step of the Edit view. So if you want to draw objects (e.g. text, stroke thickness etc.) into the Edit view at a constant size relative to the UI (e.g. constant text size on screen), you need to calculate the object’s size relative to the scale factor. See example below.
print(font.currentTab.scale) 0.414628537193 # Calculate text size desiredTextSizeOnScreen = 10 #pt scaleCorrectedTextSize = desiredTextSizeOnScreen / font.currentTab.scale print(scaleCorrectedTextSize) 24.1179733255
Type: float New in version 2.3.
-
viewPort
¶
The visible area of the Edit view in screen pixel coordinates (view coordinates).
The NSRect’s origin value describes the top-left corner (top-right for RTL, both at ascender height) of the combined glyphs’ bounding box (see
bounds
), which also serves as the origin of the view plane.The NSRect’s size value describes the width and height of the visible area.
When using drawing methods such as the view-coordinate-relative method in the Reporter Plugin, use these coordinates.
# The far corners of the Edit view: # Lower left corner of the screen x = font.currentTab.viewPort.origin.x y = font.currentTab.viewPort.origin.y # Top left corner of the screen x = font.currentTab.viewPort.origin.x y = font.currentTab.viewPort.origin.y + font.currentTab.viewPort.size.height # Top right corner of the screen x = font.currentTab.viewPort.origin.x + font.currentTab.viewPort.size.width y = font.currentTab.viewPort.origin.y + font.currentTab.viewPort.size.height # Bottom right corner of the screen x = font.currentTab.viewPort.origin.x + font.currentTab.viewPort.size.width y = font.currentTab.viewPort.origin.y
Type: NSRect New in version 2.3.
-
bounds
¶
Bounding box of all glyphs in the Edit view in view coordinate values.
Type: NSRect New in version 2.3.
-
selectedLayerOrigin
¶
Position of the active layer’s origin (0,0) relative to the origin of the view plane (see
bounds
), in view coordinates.Type: NSPoint New in version 2.3.
-
textCursor
¶
Position of text cursor in text, starting with 0.
Type: integer New in version 2.3.
-
textRange
¶
Amount of selected glyphs in text, starting at cursor position (see above).
Type: integer New in version 2.3.
-
layersCursor
¶
Position of cursor in the layers list, starting with 0.
See also
GSEditViewController.layers
Type: integer New in version 2.4.
-
direction
¶
Writing direction.
Defined constants are: LTR (left to right), RTL (right to left), LTRTTB (left to right, vertical, top to bottom e.g. Mongolian), and RTLTTB (right to left, vertical, top to bottom e.g. Chinese, Japanese, Korean)
Type: integer font.currentTab.direction = RTL
New in version 2.3.
-
features
¶
List of OpenType features applied to text in Edit view.
Type: list font.currentTab.features = ['locl', 'ss01']
New in version 2.3.
-
previewInstances
¶
Instances to show in the Preview area.
Values are
'live'
for the preview of the current content of the Edit view,'all'
for interpolations of all instances of the current glyph, or individual GSInstance objects.Type: string/GSInstance # Live preview of Edit view font.currentTab.previewInstances = 'live' # Text of Edit view shown in particular Instance interpolation (last defined instance) font.currentTab.previewInstances = font.instances[-1] # All instances of interpolation font.currentTab.previewInstances = 'all'
New in version 2.3.
-
previewHeight
¶
Height of the preview panel in the Edit view in pixels.
Needs to be set to 16 or higher for the preview panel to be visible at all. Will return 0 for a closed preview panel or the current size when visible.
Type: float New in version 2.3.
-
bottomToolbarHeight
¶
Height of the little toolbar at the very bottom of the window. Read-only.
Type: float New in version 2.4.
Functions
-
close
()¶
Close this tab.
-
saveToPDF
(path[, rect])¶
Save the view to a PDF file.
Parameters: - path – Path to the file
- rect – Optional. NSRect defining the view port. If omitted,
GSEditViewController.viewPort
will be used.
New in version 2.4.
-
GSGlyphInfo
¶
Implementation of the GSGlyphInfo object.
This contains valuable information from the glyph database. See GSGlyphsInfo
for how to create these objects.
-
class
GSGlyphInfo
¶ Properties
name
productionName
category
subCategory
components
accents
anchors
unicode
unicode2
script
index
sortName
sortNameKeep
desc
altNames
desc
Properties
-
name
¶
Human-readable name of glyph (“nice name”).
Type: unicode -
productionName
¶
Production name of glyph. Will return a value only if production name differs from nice name, otherwise None.
Type: unicode -
category
¶
This is mostly from the UnicodeData.txt file from unicode.org. Some corrections have been made (Accents, …) e.g: “Letter”, “Number”, “Punctuation”, “Mark”, “Separator”, “Symbol”, “Other”
Type: unicode -
subCategory
¶
This is mostly from the UnicodeData.txt file from unicode.org. Some corrections and additions have been made (Smallcaps, …). e.g: “Uppercase”, “Lowercase”, “Smallcaps”, “Ligature”, “Decimal Digit”, …
Type: unicode -
components
¶
This glyph may be composed of the glyphs returned as a list of
GSGlyphInfo
objects.Type: list -
accents
¶
This glyph may be combined with these accents, returned as a list of glyph names.
Type: list -
anchors
¶
Anchors defined for this glyph, as a list of anchor names.
Type: list -
unicode
¶
Unicode value
Type: unicode -
unicode2
¶
a second unicode value it present
Type: unicode -
script
¶
Script of glyph, e.g: “latin”, “cyrillic”, “greek”.
Type: unicode -
index
¶
Index of glyph in database. Used for sorting in UI.
Type: unicode -
sortName
¶
Alternative name of glyph used for sorting in UI.
Type: unicode -
sortNameKeep
¶
Alternative name of glyph used for sorting in UI, when using ‘Keep Alternates Next to Base Glyph’ from Font Info.
Type: unicode -
desc
¶
Unicode description of glyph.
Type: unicode -
altNames
¶
Alternative names for glyphs that are not used, but should be recognized (e.g., for conversion to nice names).
Type: unicode -
Methods¶
divideCurve() |
|
distance() |
|
addPoints() |
|
subtractPoints() |
|
GetOpenFile() |
|
GetSaveFile() |
|
GetFolder() |
|
Message() |
|
LogToConsole() |
|
LogError() |
-
divideCurve
(P0, P1, P2, P3, t)¶
Divides the curve using the De Casteljau’s algorithm.
param P0: | The Start point of the Curve (NSPoint) |
---|---|
param P1: | The first off curve point |
param P2: | The second off curve point |
param P3: | The End point of the Curve |
param t: | The time parameter |
return: | A list of points that represent two curves. (Q0, Q1, Q2, Q3, R1, R2, R3). Note that the “middle” point is only returned once. |
rtype: | list |
-
distance
(P0, P1)¶
calculates the distance between two NSPoints
param P0: | a NSPoint |
---|---|
param P1: | another NSPoint |
return: | The distance |
rtype: | float |
-
addPoints
(P1, P2)¶
Add the points.
param P0: | a NSPoint |
---|---|
param P1: | another NSPoint |
return: | The sum of both points |
rtype: | NSPoint |
-
subtractPoints
(P1, P2)¶
Subtracts the points.
param P0: | a NSPoint |
---|---|
param P1: | another NSPoint |
return: | The subtracted point |
rtype: | NSPoint |
-
scalePoint
(P, scalar)¶
Scaled a point.
param P: | a NSPoint |
---|---|
param scalar: | The Multiplier |
return: | The multiplied point |
rtype: | NSPoint |
-
GetSaveFile
(message=None, ProposedFileName=None, filetypes=None)¶
Opens a file chooser dialog.
param message: | |
---|---|
param filetypes: | |
param ProposedFileName: | |
return: | The selected file or None |
rtype: | unicode |
-
GetOpenFile
(message=None, allowsMultipleSelection=False, filetypes=None)¶
Opens a file chooser dialog.
param message: | A message string. |
---|---|
param allowsMultipleSelection: | |
Boolean, True if user can select more than one file | |
param filetypes: | |
list of strings indicating the filetypes, e.g., [“gif”, “pdf”] | |
param path: | The initial directory path |
return: | The selected file or a list of file names or None |
rtype: | unicode or list |
-
GetFolder
(message=None, allowsMultipleSelection = False)¶
Opens a folder chooser dialog.
param message: | |
---|---|
param allowsMultipleSelection: | |
param path: | |
return: | The selected folder or None |
rtype: | unicode |
-
Message
(title, message, OKButton=None)¶
Shows an alert panel.
param title: | |
---|---|
param message: | |
param OKButton: |
-
LogToConsole
(message)¶
Write a message to the Mac’s Console.app for debugging.
param message: |
---|
-
LogError
(message)¶
Log an error message and write it to the Macro window’s output (in red).
param message: |
---|
Constants¶
Node types
-
LINE
¶ Line node.
-
CURVE
¶ Curve node. Make sure that each curve node is preceded by two off-curve nodes.
-
QCURVE
¶ Quadratic curve node. Make sure that each curve node is preceded by at least one off-curve node.
-
OFFCURVE
¶ Off-cuve node
Export formats¶
-
OTF
¶ Write CFF based font
-
TTF
¶ Write CFF based font
-
VARIABLE
¶ Write Variable font
-
UFO
¶ Write UFO based font
-
WOFF
¶ Write WOFF
-
WOFF2
¶ Write WOFF
-
PLAIN
¶ do not package as webfont
-
EOT
¶ Write EOT
New in version 2.5.
Hint types¶
-
TOPGHOST
¶ Top ghost for PS hints
-
STEM
¶ Stem for PS hints
-
BOTTOMGHOST
¶ Bottom ghost for PS hints
-
TTANCHOR
¶ Anchor for TT hints
-
TTSTEM
¶ Stem for TT hints
-
TTALIGN
¶ Align for TT hints
-
TTINTERPOLATE
¶ Interpolation for TT hints
-
TTDIAGONAL
¶ Diagonal for TT hints
-
TTDELTA
¶ -
Delta TT hints
-
CORNER
¶ Corner Component
-
CAP
¶ Cap Component
Hint Option¶
This is only used for TrueType hints.
-
TTROUND
¶ Round to grid
-
TTROUNDUP
¶ Round up
-
TTROUNDDOWN
¶ Round down
-
TTDONTROUND
¶ Don’t round at all
-
TRIPLE = 128
Indicates a triple hint group. There need to be exactly three horizontal TTStem hints with this setting to take effect.
Callback Keys¶
This are the available callbacks
-
DRAWFOREGROUND
¶ to draw in the foreground
-
DRAWBACKGROUND
¶ to draw in the background
-
DRAWINACTIVE
¶ draw inactive glyphs
-
DOCUMENTOPENED
¶ is called if a new document is opened
-
DOCUMENTACTIVATED
¶ is called when the document becomes the active document
-
DOCUMENTWASSAVED
¶ is called when the document is saved. The document itself is passed in notification.object()
-
DOCUMENTEXPORTED
¶ if a font is exported. This is called for every instance and
notification.object()
will contain the path to the final font file.
def exportCallback(info):
try:
print(info.object())
except:
# Error. Print exception.
import traceback
print(traceback.format_exc())
# add your function to the hook
Glyphs.addCallback(exportCallback, DOCUMENTEXPORTED)
-
DOCUMENTCLOSED
¶ is called when the document is closed
-
TABDIDOPEN
¶ if a new tab is opened
-
TABWILLCLOSE
¶ if a tab is closed
-
UPDATEINTERFACE
¶ if some thing changed in the edit view. Maybe the selection or the glyph data.
-
MOUSEMOVED
¶ is called if the mouse is moved. If you need to draw something, you need to call Glyphs.redraw() and also register to one of the drawing callbacks.